Introduction to Product Categorization API
Our product categorization API is one of the most accurate e-commerce categorization AI services on the market, used by a wide range of clients, including Unicorn startups, multinational companies, online stores, ecommerce analytics platforms, adTech companies and many others.
Our API categorizes texts and URLs according to:
- Google Shopping Taxonomy with 5574 categories
- Shopify Taxonomy (latest version) with around 11k categories (GitHub Link)
- Other taxonomies, such as Amazon Shopping Categories
We also offer additional customized classifiers, adapted to your own list of categories or to other standard taxonomies.
In addition to product categorization, our Shopify API can also return Shopify Attributes based on product images. Attributes can help improve user search experience and rankings on search engines.
Example of Shopify attributes in this screenshot from our dashboard (same results are available via API):
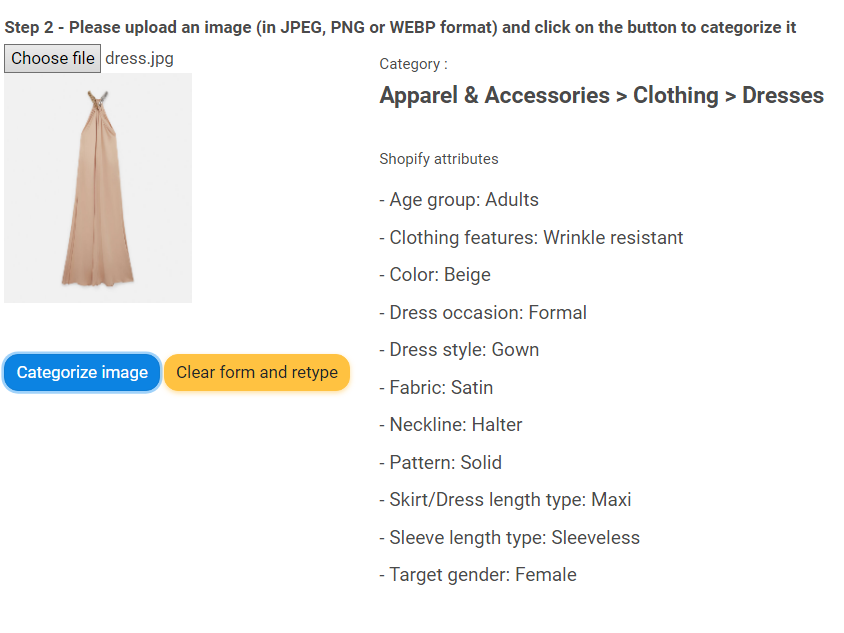
Our APIs can be used with texts, URLs and images.
Authentication
All requests should be made to endpoints that start with:
https://www.productcategorization.com/api/
You need an API key to access our API endpoints. You can obtain it by purchasing a subscription . After obtaining a plan, please log in to retrieve your API key.
Our API service expects the API key to be included in all requests as a parameter:
api_key: b4dade2ce5fb2d0b189b5eb6f0cd
HTTP responses that are successful have status code 200
. Our servers
return API data in JSON format by default.
Rules and limits
Default rate limit: 60 requests per minute. If you need a higher rate limit, please contact us.
The API returns both your total credits (total_credits
) and your remaining credits
(remaining_credits
). Pay attention to your remaining credits. If you exhaust them,
you can purchase more or upgrade your plan.
API cost:
- 1 credit per text-based API call
- 8 credits per image-based API call
Product Categorization of Texts
Our main categorization API classifies texts based on
Google Shopping Taxonomy or
Shopify Taxonomy.
Google Shopping categories (extended)
Shopify categories
The classifier also returns 10 buyer personas from our own taxonomy of 1820 buyer personas (see list here ).
Example of a request:
GET Request
https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php?query=Fluorescent Highlighters 3pc Yellow &api_key=your_api_key
You can also pass your API key in the header:
curl -X GET "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get_bearer.php?query=your_query" \
-H "X-API-KEY: api"
Query Parameters
Parameter | Type | Description |
---|---|---|
query | string | Text to be categorized |
api_key | string | Your API key |
confidence | string | set this parameter to 1, if you want personas returned together with confidence scores for each persona (by default only personas are returned). Not using this parameter or using 0 with return personas without confidence scores. |
expand_context | string | set this parameter to 1 if your query text is short or ambiguous. Our AI engine will then generate the description of product and use it for classification. Note that it is not necessary to use this option if your product titles/descriptions are already clear and unambigious. If not setting this parameter in your call or setting it to 0, the expansion will be bypassed. |
The above command returns response in JSON format, structured like this (ID is Google Shopping Category ID):
{
"total_credits": 100044,
"remaining_credits": 33075,
"language": "en",
"classification": "Office Supplies > Office Instruments > Writing & Drawing Instruments",
"buyer_personas": [
"Business Professional",
"Office Professional",
"Administrative Coordinator",
"Busy Office Manager",
"Creative Professional",
"Efficient Office Manager",
"School Supplies Shopper",
"Efficient Organizer",
"Document Specialist",
"Professional Secretary"
],
"buyer_personas_confidence_selection": {
"Office Professional": 0.9,
"Business Professional": 0.8,
"Document Specialist": 0.8,
"Administrative Coordinator": 0.7,
"Efficient Office Manager": 0.7,
"Professional Secretary": 0.7,
"Busy Office Manager": 0.6,
"Efficient Organizer": 0.6,
"Creative Professional": 0.5,
"School Supplies Shopper": 0.4
},
"ID": "977",
"status": 200
}
Example response when using confidence=1 and expand_context=1:
{
"total_credits": 100044,
"remaining_credits": 32957,
"text_for_expanded_context": "Fluorescent Highlighters 3pc Yellow ",
"expanded_context": "**Title: Fluorescent Highlighters - 3-Pack Yellow**\n\n**Expanded Description:**\n\nIlluminate your notes and enhance your study sessions
with our Fluorescent Highlighters in a vibrant yellow hue. This 3-pack of high-quality highlighters is designed to make your important information stand out,
ensuring that you never miss a key point again. Each highlighter features a chisel tip that allows for versatile use, whether you need to underline, highlight,
or create bold accents in your text. \n\nThe bright yellow ink is not only eye-catching but also quick-drying, preventing smudging and
allowing for immediate handling of your documents. Ideal for students, professionals, and anyone who values organization, these highlighters are perfect
for textbooks, planners, reports, and more. The ergonomic design ensures a comfortable grip, making it easy to highlight for
extended periods without fatigue.\n\nThese fluorescent highlighters are also non-toxic and safe for everyday use, making them suitable for all ages. Whether you're preparing for exams, organizing your work, or simply want to add a splash of color to your notes, this 3-pack of yellow highlighters is an essential tool for effective studying and efficient information retention. Brighten up your workspace and make your marking tasks a breeze with these reliable highlighters!",
"language": "en",
"classification": "Office Supplies > Office Instruments > Writing & Drawing Instruments > Markers & Highlighters > Highlighters",
"buyer_personas": [
"Administrative Coordinator",
"Corporate Office Manager",
"Office Professional",
"Efficient Office Manager",
"Professional Stationery Specialist",
"Creative Professional",
"Busy Office Manager",
"Home Office Enthusiast",
"School Supplies Shopper"
],
"buyer_personas_confidence_all": {
"Student": 0.9,
"Creative Learning Advocate": 0.8,
"Educational Institution Supplier": 0.8,
"School Supplies Shopper": 0.8,
"Family Meal Planner": 0.7,
"Professional Writer": 0.7,
"Digital Marketing Professional": 0.7,
"Health Conscious Consumer": 0.7,
"Professional Secretary": 0.6,
"Corporate Communications Specialist": 0.6,
"Event Professional": 0.6,
"Professional Stylist": 0.6,
"Trendy Home Stylist": 0.6,
"Professional Artist": 0.6,
"Professional Illustrator": 0.6,
"Professional Transcriptionist": 0.6,
"Professional Editor": 0.6,
"Home Decor Enthusiast": 0.6,
"DIY Decorator": 0.6,
"Trendy Gift Buyer": 0.6,
"Trendy Fashionista": 0.6,
"Trendy Decorator": 0.6,
"Creative Parent": 0.6,
"Art Enthusiast Parent": 0.6,
"Family Fun Enthusiast": 0.6,
"Gift Shop Owner": 0.6,
"Unique Gift Seeker": 0.6,
"Luxury Gift Shopper": 0.6,
"Trendy Toy Hunter": 0.6,
"Trendy Teen": 0.6,
"Trendy Businessperson": 0.6,
"Trendy Night Owl": 0.6,
"Trendy Gadget Fan": 0.6,
"Trendy Accessory Enthusiast": 0.6,
"Trendy Apartment Dweller": 0.6,
"Trendy Decor Aficionado": 0.6,
"Trendy Outdoor Enthusiast": 0.6,
"Trendy Home Designer": 0.6,
"Trendy Event Planner": 0.6
},
"buyer_personas_confidence_selection": {
"Administrative Coordinator": 0.8,
"Corporate Office Manager": 0.7,
"Office Professional": 0.9,
"Efficient Office Manager": 0.8,
"Professional Stationery Specialist": 0.9,
"Creative Professional": 0.6,
"Busy Office Manager": 0.7,
"Home Office Enthusiast": 0.5,
"School Supplies Shopper": 1
},
"ID": "543609",
"status": 200
}
Curl Request
Use Curl with your API key to get the classification:
curl --request GET 'https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php?query=Fluorescent Highlighters 3pc Yellow &api_key=your_api_key'
Example Code
Below are minimal code examples for making requests to our API in different programming languages.
Example Code in Python
import requests
from urllib.parse import quote_plus
api_base_url = "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php?"
api_key = "your_api_key"
query_text = "Fluorescent Highlighters 3pc Yellow"
encoded_query_text = quote_plus(query_text)
final_url = f"{api_base_url}query={encoded_query_text}&api_key={api_key}"
response = requests.get(final_url)
print(response.text)
Example Code in JavaScript
const apiBaseUrl = "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php?";
const apiKey = "your_api_key";
const queryText = "Fluorescent Highlighters 3pc Yellow";
const encodedQueryText = encodeURIComponent(queryText);
const finalUrl = `${apiBaseUrl}query=${encodedQueryText}&api_key=${apiKey}`;
fetch(finalUrl)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error('Error:', error));
Example Code in Ruby
require 'uri'
require 'net/http'
api_base_url = "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php"
api_key = "your_api_key"
query_text = "Fluorescent Highlighters 3pc Yellow"
encoded_query = URI.encode_www_form_component(query_text)
url = URI("#{api_base_url}?query=#{encoded_query}&api_key=#{api_key}")
response = Net::HTTP.get(url)
puts response
Example Code in PHP
$api_base_url = "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php";
$api_key = "your_api_key";
$query_text = "Fluorescent Highlighters 3pc Yellow";
$query_text_encoded = urlencode($query_text);
$final_url = $api_base_url . "?query=" . $query_text_encoded . "&api_key=" . $api_key;
$response = file_get_contents($final_url);
echo $response;
Example Code in C#
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program {
static async Task Main(string[] args) {
var apiBaseUrl = "https://www.productcategorization.com/api/ecommerce/ecommerce_category6_get.php?";
var apiKey = "your_api_key";
var queryText = "Fluorescent Highlighters 3pc Yellow";
var encodedQueryText = Uri.EscapeDataString(queryText);
var finalUrl = $"{apiBaseUrl}query={encodedQueryText}&api_key={apiKey}";
using (HttpClient client = new HttpClient()) {
var response = await client.GetStringAsync(finalUrl);
Console.WriteLine(response);
}
}
}
Product Categorization of Images
Our categorization and attributes API can classify images based on Shopify Taxonomy: https://github.com/Shopify/product-taxonomy/tree/main
Below is a Python example for calling our image-categorization endpoint in case of using URL for image (modify the code accordingly in case of using local image file):
import requests
import io
def call_api(image_url, text, ip='0', api_key='your_api_key', login='0'):
api_endpoint = 'https://www.productcategorization.com/api/ecommerce/ecommerce_shopify_image.php'
# Download the image
response = requests.get(image_url)
if response.status_code != 200:
return {'error': 'Failed to download image'}
# Create a file-like object
image_file = io.BytesIO(response.content)
# Prepare the data
data = {
'ip': ip,
'api_key': api_key,
'login': login,
'text': text
}
files = {
'image': ('image.jpg', image_file, 'image/jpeg')
}
try:
response = requests.post(api_endpoint, data=data, files=files)
response.raise_for_status()
return response.json()
except requests.exceptions.RequestException as e:
return {'error': f'API call failed: {str(e)}'}
Errors
The API returns a status
value for each request.
For example, a successful request might look like:
{
"classification": "...",
"status": 200,
...
}
In case of an error, the status code can be interpreted by the following table:
Error Code | Meaning |
---|---|
200 | Request was successful. |
400 | Error in forming request. Check documentation. |
401 | Invalid API key. Purchase a subscription or check key spelling. |
403 | Monthly quota exhausted. Upgrade plan or add credits. |